Efficient memory management is one of the cornerstones of game development. Without proper memory handling, even the most innovative games can suffer from performance issues, crashes, or dreaded memory leaks. This blog post delves into essential techniques and tips for managing memory in game development, ensuring your game runs smoothly and efficiently.
Why Memory Management Matters in Game Development
Games are resource-intensive applications. They juggle large assets, complex algorithms, and dynamic user interactions in real time. Poor memory management can result in:
- Memory Leaks: Unused memory not being released, leading to increased memory usage over time.
- Performance Drops: Excessive memory allocation or fragmentation causing slowdowns.
- Crashes: Insufficient memory availability or heap corruption.
By understanding and optimizing memory usage, developers can create games that are both reliable and performant.
Key Concepts in Game Memory Management
Before diving into techniques, let’s explore some fundamental concepts:
- Stack vs. Heap Memory:
- Stack: Used for static memory allocation and function call management. It’s fast but limited in size.
- Heap: Used for dynamic memory allocation, offering flexibility at the cost of slower access.
- Garbage Collection:
- Some programming languages like C# (used in Unity) handle memory automatically through garbage collection. While convenient, this can introduce performance spikes if not managed properly.
- Manual Memory Management:
- In languages like C++, developers manually allocate and deallocate memory using commands like
new
anddelete
. This offers control but increases the risk of errors.
- In languages like C++, developers manually allocate and deallocate memory using commands like
Tips for Effective Game Memory Management
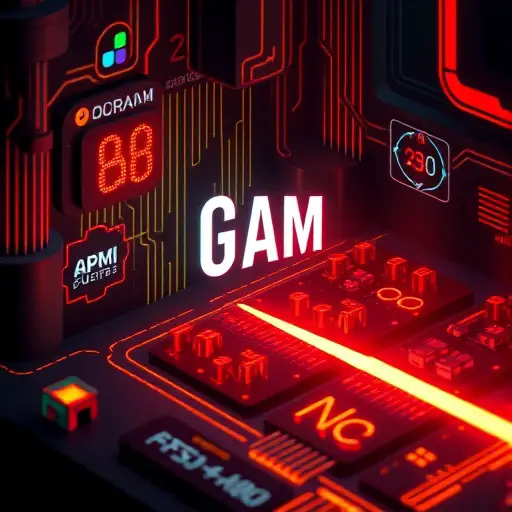
1. Minimize Dynamic Allocations
Dynamic memory allocation at runtime can slow down performance. Use these strategies to minimize it:
- Object Pools: Preallocate a pool of objects that can be reused instead of creating and destroying objects frequently. For example, in a shooting game, bullets can be reused from a pool rather than instantiated and destroyed for each shot.
- Static Allocation: Where possible, allocate memory at the start of a level or game.
2. Optimize Asset Loading
Large textures, models, and audio files can consume significant memory. Consider these optimizations:
- Lazy Loading: Load assets only when needed. For instance, load textures for a boss character only when the player enters the boss room.
- Compression: Use compressed formats for textures and audio to reduce memory usage.
- Streaming: Stream assets dynamically during gameplay instead of loading them all at once.
3. Avoid Fragmentation
Memory fragmentation occurs when free memory is broken into smaller chunks, making it difficult to allocate large contiguous blocks.
- Use Memory Pools: Allocate fixed-size blocks of memory to reduce fragmentation.
- Align Memory Usage: Keep memory allocations aligned to improve cache performance and reduce fragmentation.
4. Detect and Prevent Memory Leaks
Memory leaks occur when allocated memory isn’t properly deallocated. To avoid this:
- Track Allocations: Use tools or custom memory managers to log and monitor allocations.
- Smart Pointers: In C++, use smart pointers (
std::unique_ptr
,std::shared_ptr
) to automate memory management. - Testing and Profiling: Regularly test for leaks using tools like Valgrind (C++) or Unity’s Profiler.
5. Manage Garbage Collection
For garbage-collected languages like C#:
- Reduce Allocations: Minimize object creation to reduce garbage collection frequency.
- Manual Triggers: Trigger garbage collection during non-critical game moments, like loading screens.
- Avoid Long-Lived Objects: Objects that persist unnecessarily can hinder garbage collection efficiency.
6. Use Custom Memory Managers
For games with complex memory requirements, custom memory managers provide greater control:
- Stack Allocators: Manage memory in a LIFO (last in, first out) manner, perfect for temporary allocations.
- Frame Allocators: Allocate memory per frame and clear it at the end of each frame, useful for temporary data.
- Slab Allocators: Allocate fixed-size chunks for uniform object types.
Use Case Scenarios
Scenario 1: Optimizing a Mobile Game
A mobile RPG is experiencing frequent crashes on older devices due to high memory usage. By implementing asset streaming and object pooling, the game’s memory footprint is reduced significantly, preventing crashes and improving performance.
Scenario 2: Preventing Memory Leaks in a Multiplayer Game
A multiplayer shooter game faces performance degradation over long sessions. Profiling reveals memory leaks in object instantiation for projectiles. Switching to an object pool eliminates the leaks, maintaining smooth gameplay.
Scenario 3: Enhancing VR Game Performance
A VR game suffers from performance spikes due to frequent garbage collection. By preallocating memory and batching allocations, garbage collection frequency is reduced, leading to a smoother VR experience.
Tools for Memory Management in Game Development
- Unity Profiler: Analyze memory usage, detect leaks, and optimize performance.
- Unreal Engine Insights: Monitor memory allocation and fragmentation in Unreal Engine projects.
- Valgrind: A powerful tool for detecting memory leaks and errors in C++.
- Custom Debugging Tools: Build tools tailored to your game’s specific needs to log and analyze memory usage.
Conclusion
Memory management is a critical aspect of game development. By understanding and implementing the techniques discussed in this post, developers can ensure their games run smoothly, avoid crashes, and deliver a seamless experience for players. Whether you’re working on a casual mobile game or an expansive AAA title, efficient memory management should always be a priority.