Artificial Intelligence (AI) has become a crucial element in modern game development. From enemies that react to player actions to NPCs with intricate behaviors, AI enhances the overall gameplay experience. One of the most widely used techniques for implementing game AI is the behavior tree. Especially in Unity, behavior trees offer a flexible and scalable way to manage complex AI behaviors.
In this post, we’ll dive deep into the concept of behavior trees in Unity, their pros and cons, and provide a detailed use case to show how they work in a real-world scenario.
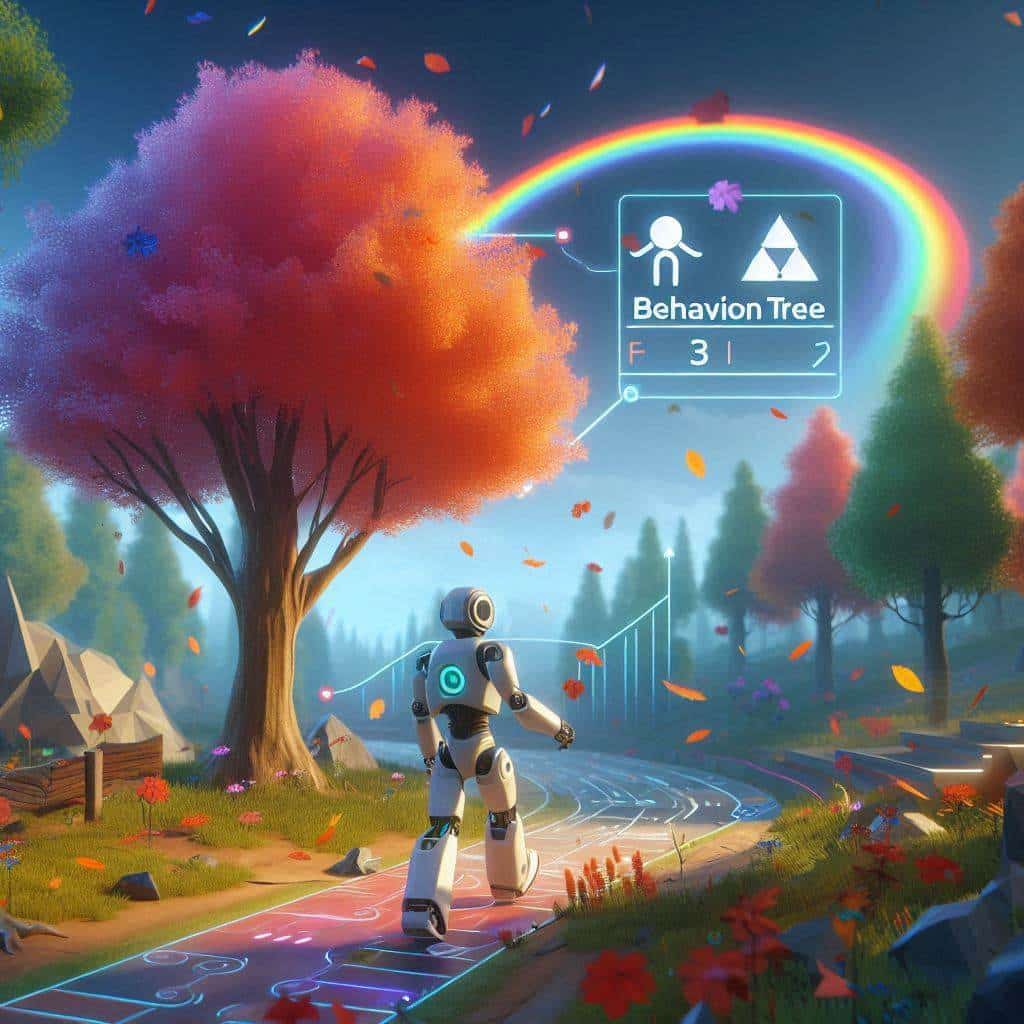
What Is a Behavior Tree?
A behavior tree (BT) is a hierarchical model used to define the behaviors of AI entities. It breaks down complex tasks into manageable actions, allowing game characters to make decisions based on specific conditions.
Behavior trees consist of nodes, which represent either actions or decisions. These nodes are connected in a tree-like structure, making it easy to follow the AI’s decision-making process. The main benefit of a behavior tree is its modular nature. You can break down complicated tasks into smaller, more manageable parts, which makes it easier to understand and control how your game characters behave.
Key Components of a Behavior Tree
Before we jump into the specifics, let’s break down the primary components of a behavior tree:
- Root Node: The entry point of the tree where execution begins.
- Composite Nodes: These are decision-making nodes that determine which branch of the tree to execute.
- Sequence: Executes child nodes in order; if one fails, the whole sequence fails.
- Selector: Executes child nodes until one succeeds.
- Decorator Nodes: Modify the behavior of a node, typically adding conditions like looping or checking a condition before execution.
- Leaf Nodes: The actions or tasks the AI performs, such as moving toward a target or attacking.
Implementing Behavior Trees in Unity
Unity offers several ways to implement behavior trees, whether through custom code or using pre-built assets from the Unity Asset Store. If you’re just starting out, the Unity Asset Store provides several excellent tools for creating behavior trees, including the popular Behavior Designer and NodeCanvas.
Custom Implementation
If you prefer more control, you can implement behavior trees manually in Unity using C#. Here’s a simple example of how to create a basic behavior tree for an enemy AI:
public class BehaviorTree : MonoBehaviour
{
public enum NodeState { RUNNING, SUCCESS, FAILURE }
public abstract class Node
{
public abstract NodeState Evaluate();
}
public class Sequence : Node
{
private List<Node> nodes = new List<Node>();
public Sequence(List<Node> nodes) { this.nodes = nodes; }
public override NodeState Evaluate()
{
foreach (Node node in nodes)
{
NodeState state = node.Evaluate();
if (state == NodeState.FAILURE)
return NodeState.FAILURE;
else if (state == NodeState.RUNNING)
return NodeState.RUNNING;
}
return NodeState.SUCCESS;
}
}
// Example of other nodes can be added here.
}
This sample demonstrates the structure of a sequence node in a behavior tree. The AI will go through each child node, and the sequence only succeeds if all children succeed.
Using Assets from the Unity Asset Store
If coding a behavior tree from scratch feels daunting, Unity’s Behavior Designer is a fantastic tool that simplifies the process of creating AI. It allows you to visually construct behavior trees without writing much code.
Steps to use Behavior Designer:
- Download the plugin from the Unity Asset Store.
- Create a behavior tree using its node-based editor.
- Add custom scripts or use pre-defined actions to manage character behaviors.
Pros of Using Behavior Trees in Unity
1. Modularity and Reusability
Behavior trees allow you to break complex behavior into smaller, reusable components. Once a behavior is written, it can be reused in multiple AI agents with little modification.
2. Scalability
As your game grows, so do the needs of your AI. Behavior trees allow you to add new behaviors without completely overhauling the system. Simply create new nodes or modify existing ones.
3. Visual Representation
Many developers favor behavior trees for their visual nature. Tools like Behavior Designer allow you to visualize the flow of your AI’s logic, making debugging and iteration much more intuitive.
4. Simplicity in Managing Complex Behaviors
Unlike finite state machines (FSM), which can become overly complex as states increase, behavior trees remain easy to manage and understand, even with a large number of nodes.
5. Non-Blocking Execution
Behavior trees allow AI to switch between different behaviors seamlessly. If an action fails, the AI can quickly pivot to another behavior, leading to more dynamic and reactive game characters.
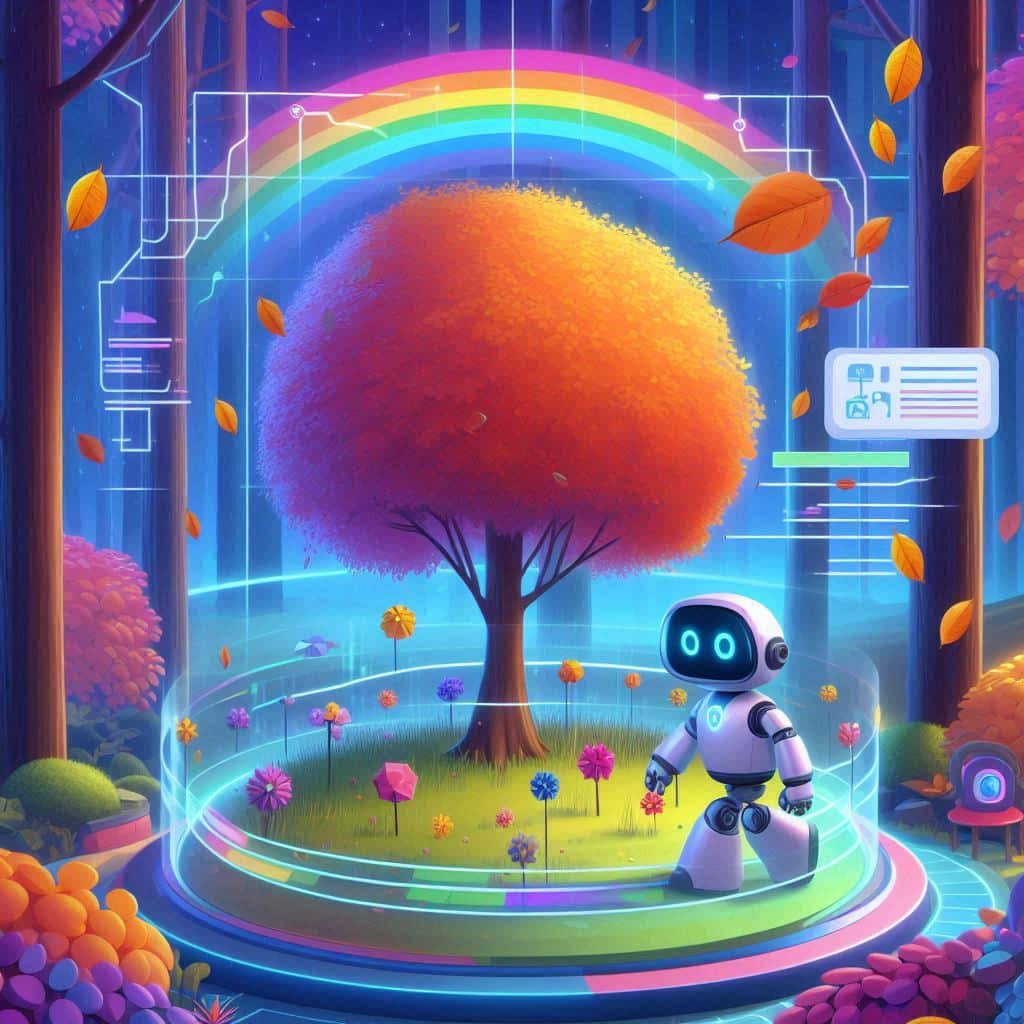
Cons of Using Behavior Trees in Unity
1. Learning Curve
For developers new to behavior trees, there can be a steep learning curve. Understanding how to structure the tree efficiently takes time, especially when dealing with decorators and complex node types.
2. Performance Overhead
For very large and complex behavior trees, there can be some performance concerns, particularly on resource-constrained platforms like mobile devices. However, this can often be mitigated with good optimization practices.
3. Harder to Debug at Scale
While behavior trees offer a great visual representation of AI logic, they can become cumbersome as they scale. A large behavior tree with numerous nodes may become difficult to manage, even with a visual editor.
Use Case: Behavior Tree in a Stealth Game
Let’s look at a stealth game example to better understand the practical use of behavior trees in Unity.
Scenario:
In this game, you control a spy who must avoid being detected by patrolling guards. The guards have different states, such as patrolling, chasing the player, and returning to their post when the player is lost.
Behavior Tree Breakdown:
- Root Node: Entry point for the AI guard.
- Sequence Node: Guards first patrol an area. If they spot the player, they enter a chase sequence.
- Selector Node: The guard first checks for the player’s presence. If the player is spotted, it moves into attack mode. If not, it continues to patrol.
- Leaf Node: Leaf nodes control the specific actions the guard takes, such as walking a patrol route, chasing the player, or returning to their post.
Here’s how the tree would look:
- Patrol Sequence:
- Walk from point A to point B.
- Check if the player is in sight.
- Chase Sequence:
- If the player is spotted, begin chasing them.
- If the player hides or escapes, return to patrol.
- Attack Sequence:
- If the player is within range, attack.
This simple example shows how behavior trees make it easy to manage different actions that depend on the current state of the game world.
Pros and Cons Recap
Pros
- Modularity and Reusability: Nodes can be reused across different AI characters.
- Scalability: Easy to expand behaviors as the game grows.
- Visual Representation: Tools like Behavior Designer simplify the process.
- Dynamic and Reactive AI: Seamless switching between behaviors.
- Non-Blocking Execution: More flexible than finite state machines.
Cons
- Learning Curve: May be difficult for beginners to master.
- Performance: Large trees can slow down performance on mobile devices.
- Complexity: Debugging large behavior trees can be challenging.
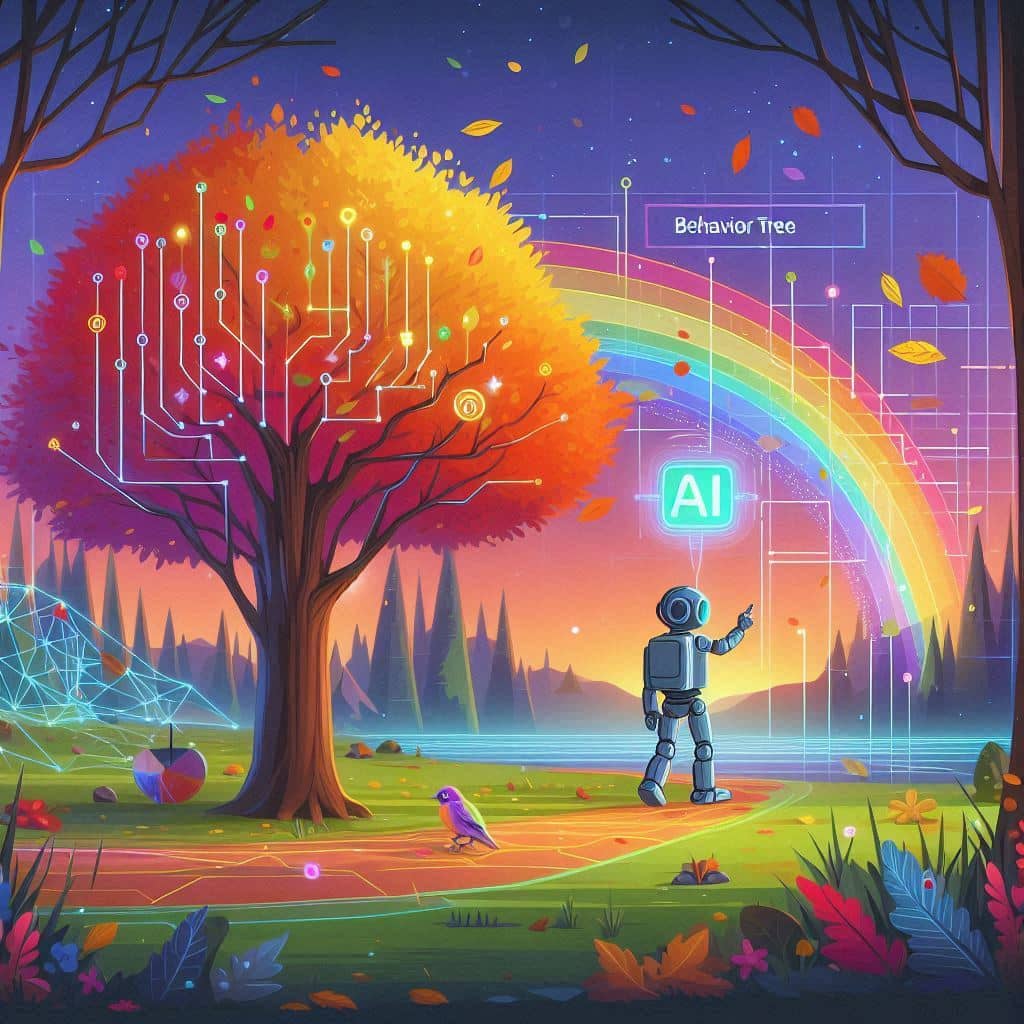
Conclusion
Behavior trees are a powerful tool for creating complex, responsive AI in games, particularly when using Unity. While there are some learning curves and potential performance concerns, the scalability, modularity, and visual structure of behavior trees make them an excellent choice for game AI.
Whether you choose to implement your own behavior tree from scratch or use one of the many assets available in the Unity Asset Store, understanding how to structure your AI’s behavior with this method will greatly enhance your game’s interactivity.